Svelte Avatar - Flowbite
Use the avatar component to show a visual representation of a user profile using an image element or SVG object based on multiple styles and sizes
The avatar component can be used as a visual identifier for a user profile on your website and you can use the examples from Flowbite to modify the styles and sizes of these components using the utility classes from Tailwind CSS.
Setup #
Import Avatar
.
If you are using the user dropdown, import Dropdown
, DropdownHeader
, DropdownItem
, DropdownDivider
.
If you are using tooltip for avatar import Tooltip
.
<script>
import { Avatar, Dropdown, DropdownHeader, DropdownItem, DropdownDivider, Tooltip } from "flowbite-svelte"
</script>
Default avatar #
Use this example to create a circle and rounded avatar on an image element.
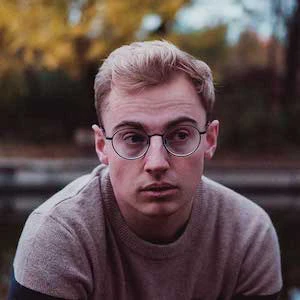
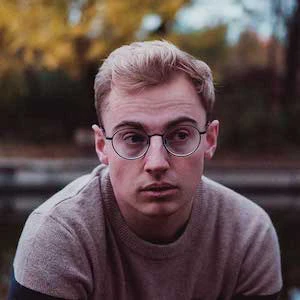
<div class="flex space-x-4"> <Avatar src="/images/profile-picture-2.webp"/> <Avatar src="/images/profile-picture-2.webp" rounded/> </div>
Bordered #
You can apply a border around the avatar component.
If you can use the ring-{color}
class from Tailwind CSS to modify ring color.
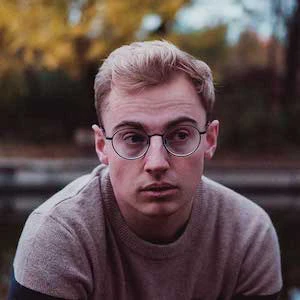
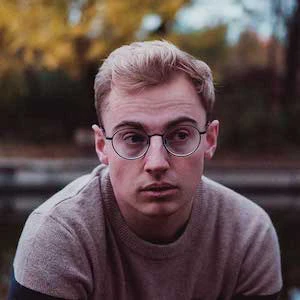
<Avatar src="/images/profile-picture-2.webp" border/> <Avatar src="/images/profile-picture-2.webp" border class="ring-red-400 dark:ring-red-300"/>
Placeholder #
When there is no custom image available a placehoder is displayed.
<Avatar /> <Avatar rounded /> <Avatar border /> <Avatar rounded border />
Placeholder initials #
This example can be used to show the initials of the user’s first and last name as a placeholder when no profile picture is available.
<Avatar>JL</Avatar>
Avatar tooltip #
Use this example to show a tooltip when hovering over the avatar.
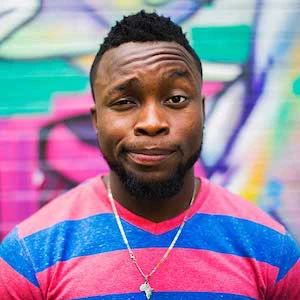
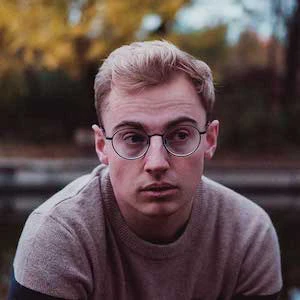
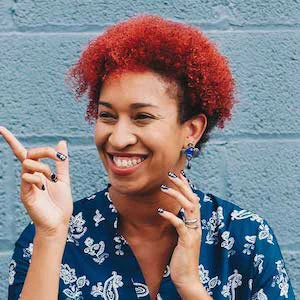
<script> import {Avatar, Tooltip} from 'flowbite-svelte' </script> <Avatar data-name="Jese Leos" rounded src="/images/profile-picture-1.webp"/> <Avatar data-name="Robert Gouth" rounded src="/images/profile-picture-2.webp"/> <Avatar data-name="Bonnie Green" rounded src="/images/profile-picture-3.webp"/> <Tooltip triggeredBy="[data-name]" on:show={e => name = e.target.dataset.name}>{name}</Tooltip>
Dot indicator #
Use a dot element relative to the avatar component as an indicator for the user (eg. online or offline status).
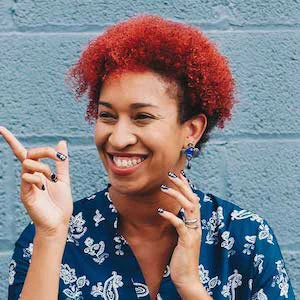
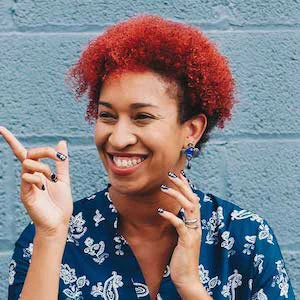
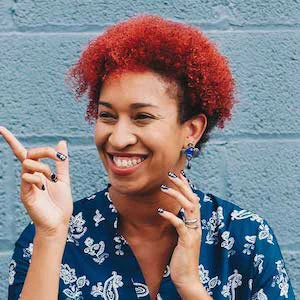
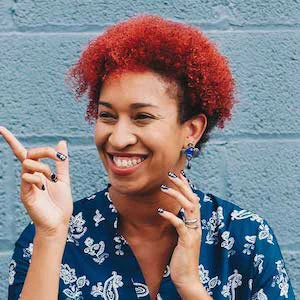
<Avatar src="/images/profile-picture-3.webp" dot={{top:true, color:"bg-red-400"}}/> <Avatar src="/images/profile-picture-3.webp" dot={{top:true, color:"bg-red-400"}} rounded /> <Avatar src="/images/profile-picture-3.webp" dot={{color:"bg-green-400"}}/> <Avatar src="/images/profile-picture-3.webp" dot={{color:"bg-green-400"}} rounded/>
Stacked #
Use this example if you want to stack a group of users by overlapping the avatar components.
<div class="flex mb-5"> <Avatar src="/images/profile-picture-1.webp" stacked/> <Avatar src="/images/profile-picture-2.webp" stacked /> <Avatar src="/images/profile-picture-3.webp" stacked /> <Avatar stacked /> </div> <div class="flex"> <Avatar src="/images/profile-picture-1.webp" stacked /> <Avatar src="/images/profile-picture-2.webp" stacked /> <Avatar src="/images/profile-picture-3.webp" stacked /> <Avatar stacked href="/" class="bg-gray-700 text-white hover:bg-gray-600">+99</Avatar> </div>
Avatar text #
This example can be used if you want to show additional information in the form of text elements such as the user’s name and join date.
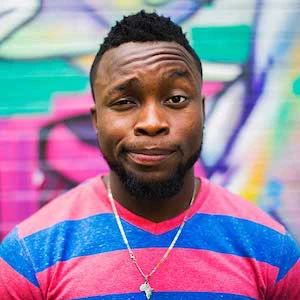
<div class="flex items-center space-x-4"> <Avatar src="/images/profile-picture-1.webp" rounded/> <div class="space-y-1 font-medium dark:text-white"> <div>Jese Leos</div> <div class="text-sm text-gray-500 dark:text-gray-400">Joined in August 2014</div> </div> </div>
User dropdown #
Use this example if you want to show a dropdown menu when clicking on the avatar component.
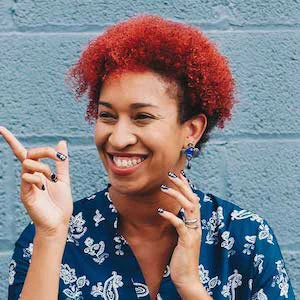
<script> import {Avatar, Dropdown, DropdownHeader, DropdownItem, DropdownDivider} from 'flowbite-svelte' </script> <Avatar id="user-drop" src="/images/profile-picture-3.webp" dot={{color:'bg-green-400'}} /> <Dropdown triggeredBy="#user-drop"> <DropdownHeader> <span class="block text-sm"> Bonnie Green </span> <span class="block truncate text-sm font-medium"> name@flowbite.com </span> </DropdownHeader> <DropdownItem>Dashboard</DropdownItem> <DropdownItem>Settings</DropdownItem> <DropdownItem>Earnings</DropdownItem> <DropdownDivider /> <DropdownItem>Sign out</DropdownItem> </Dropdown>
Sizes #
Select size from xs | sm | md | lg | xl.
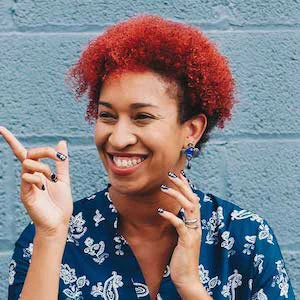
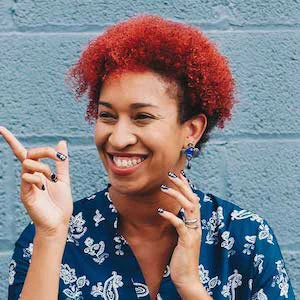
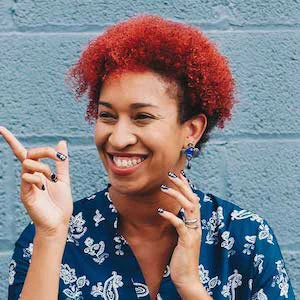
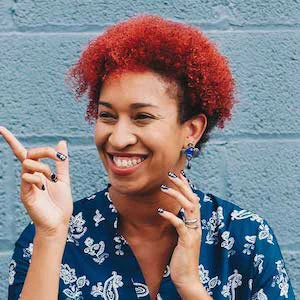
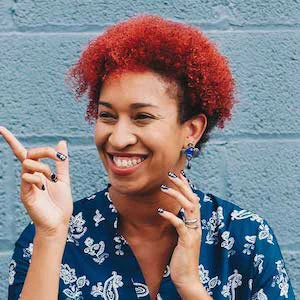
<div class=" flex flex-wrap justify-center space-x-4"> <Avatar src="/images/profile-picture-3.webp" rounded size="xs" /> <Avatar src="/images/profile-picture-3.webp" rounded size="sm" /> <Avatar src="/images/profile-picture-3.webp" rounded size="md" /> <Avatar src="/images/profile-picture-3.webp" rounded size="lg" /> <Avatar src="/images/profile-picture-3.webp" rounded size="xl" /> </div>
Props #
The component has the following props, type, and default values. See types page for type information.
Name | Type | Default |
---|---|---|
src | string | '' |
href | string | '#' |
rounded | boolean | false |
border | boolean | false |
stacked | boolean | false |
dot | DotType | { top: false, color: 'bg-gray-300 dark:bg-gray-500' } |
alt | string | '' |
size | 'xs' | 'sm' | 'md' | 'lg' | 'xl' | 'md' |